java编程学习:数组的基本用法
数组是一组类型相同的数据的集合,数组中的每个数据称为元素。在Java中,数组本身也是Java对象。数组中的元素可以是任意类型(包括基本类型和引用类型),但同一个数组里只能存放类型相同的元素。
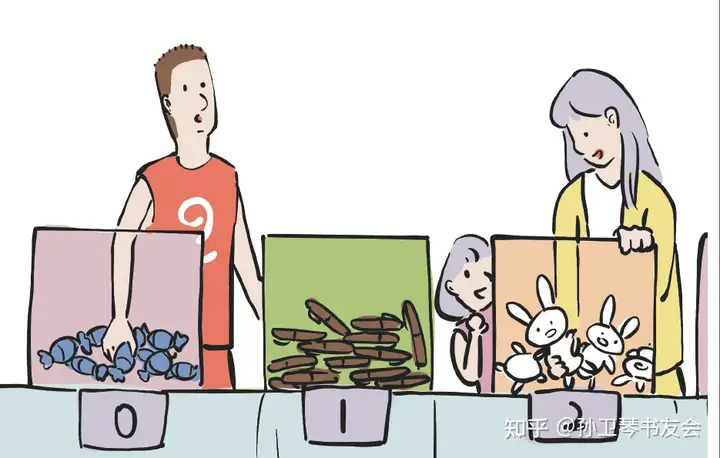
创建数组包括如下步骤。
(1)声明一个数组类型的引用变量,简称为数组变量。
(2)用new语句创建数组的实例。new语句为数组分配内存,并且为数组中的每个元素赋予默认值。
(3)初始化,即为数组的每个元素设置合适的初始值。
1. 数组变量的声明
以下代码声明了两个引用变量scores和names,它们分别为int数组类型和String数组类型:
int[] scores; //scores数组存放int类型的数据
String[] names; //names数组存放String类型的数据
以下数组变量的声明方式也是合法的:
int scores[];
String names[];
声明数组变量的时候,不能指定数组的长度,以下声明方式是非法的:
int scores[50]; //非法
int names[50]; //非法
2. 创建数组对象
数组对象和其他Java对象一样,也用new语句创建,例如:
//创建一个int类型数组,存放50个int数据
int[] scores=new int[50];
在用new语句创建数组对象时,需要指定数组长度,数组长度表示数组中包含的元素数目。
new语句执行以下步骤。
(1)在内存中为数组分配内存空间,以上代码创建了一个包含50个元素的int类型数组。每个元素都是int类型,占4个字节,因此整个数组对象在内存中占用200个字节。
(2)为数组中的每个元素赋予其数据类型的默认值。以上int数组中的每个元素都是int类型,因此它们的默认值都为0。
在以下程序代码中,scores[0]表示scores数组中的第一个元素,它是int类型,默认值为0; names[0]表示names数组中的第一个元素,它是String类型,默认值为null:
int[] scores=new int[50];
System.out.println(scores[0]); //打印0
String[] names=new String[50];
System.out.println(names[0]); //打印null
(3)返回数组对象的引用。
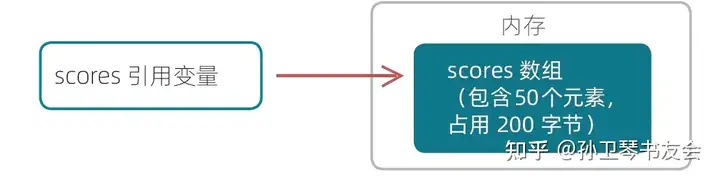
3. 访问数组的元素和长度
数组中的每个元素都有一个索引,或者称为下标。数组中的第一个元素的索引为0,第二个为1,依次类推。以下程序代码创建了一个长度为4的colors字符串数组,colors[0]表示第一个元素,colors[1]表示第二个元素:
String[] colors=new String[4];
colors[0]="Red"; //把colors数组的第一个元素设为“Red”
System.out.println(colors[0]); //打印Red
System.out.println(colors[1]); //打印null
//抛出ArrayIndexOutOfBoundsException异常
System.out.println(colors[4]);
在colors数组中,最后一个元素为colors[3],如果访问colors[4],由于索引超出了colors数组的边界,运行时会抛出ArrayIndexOutOfBoundsException运行时异常。这种异常是由于程序代码中的错误引起的,应该在调试阶段通过改进程序代码来消除它们。
所有Java数组都有一个length属性,表示数组的长度,它的声明形式为:
public final length;
length属性被public和final修饰,因此在程序中可以读取数组的length属性,但不能修改这一属性,例如:
int[] days=new int[31];
System.out.println(days.length); //打印31
days.length=10; //非法,length属性为final类型,不能被修改
4. 数组的初始化
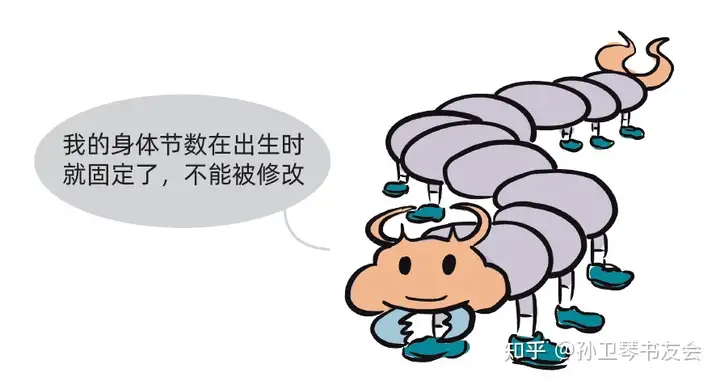
数组被创建后,每个元素被自动赋予其数据类型的默认值。另外,还可以在程序中对数组元素显式初始化。例如:
int[] x= new int[5]; //创建数组
for(int i=0; i<x.length;i++) //初始化数组x
x[i]=x.length-i;
为了简化编程,也可以按如下方式创建并初始化数组:
//创建并初始化数组
int[] x= new int[]{5, 4, 3, 2, 1};
char[] y= new char[] {'a','b','c','d'};
String[] z={"Monday","Tuesday"};
以下是非法的数组初始化方式:
//非法,不能在[]中指定数组的长度
int[] x= new int[5]{5, 4, 3, 2, 1};
5. 遍历访问数组
大力:“数组中元素的索引从0开始编号,如何通过循环语句来遍历访问数组中的每个元素呢?”
卫琴:“以scores数组为例,scores[i]表示索引为i的元素。在循环流程中,只要让变量i从0递增到scores.length-1,就可以访问scores数组的每个元素。”
例程1的ScoreTool类的max()方法会通过循环语句遍历访问scores数组,从中找到取值最大的元素。
例程1 ScoreTool.java
public class ScoreTool{
public static int max(int[] scores){
int result=0;
for(int i=0;i<scores.length; i++){
if(i==0)
result=scores[i];
else if(scores[i]>result)
result=scores[i];
}
return result;
}
public static void main(String[] args){
int[] scores={89,96,84,67,55} ;
System.out.println(max(scores)); //打印96
}
}